The Unspoken Rules of Coding for Both Novice and Sage Developers
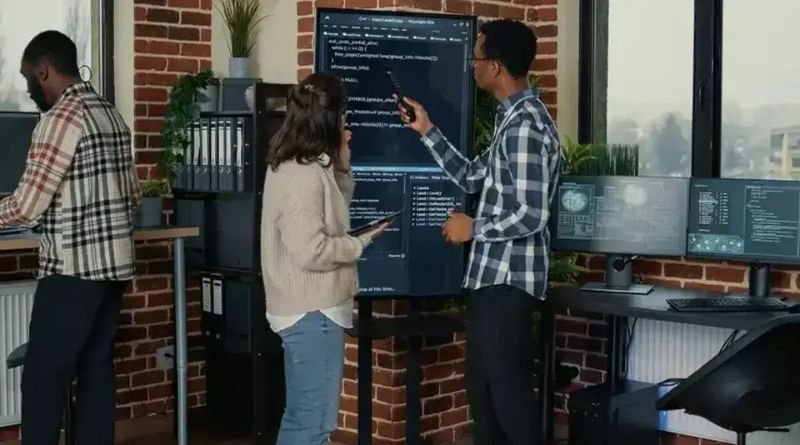
Coding, whether you’re just starting out or have years of experience under your belt, is more than just writing lines of code. It’s a craft that requires creativity, patience, and an understanding of unwritten rules that can greatly impact how efficient and effective you are as a developer. These unspoken rules often stem from best practices, community standards, and common sense, and while they might not always be part of official documentation, adhering to them can significantly improve your coding experience. This article aims to explore these unspoken rules of coding, applicable to both novice and sage developers, to help you become a better programmer and collaborator.
Table of Contents
1. Keep Your Code Simple and Readable
Simplicity is a virtue in coding. Whether you’re just beginning or well-seasoned, keeping your code as simple and readable as possible is crucial. Avoid complex and overly nested code whenever you can. Use meaningful variable and function names, comment your code to explain logic where necessary, and strive to write concise code that is easy to understand. This not only benefits you as you revisit and maintain your code in the future but also makes it easier for others—whether teammates or reviewers—to understand and collaborate on your work.
Example:
def calculate_area(radius):
return 3.14159 * radius * radius
instead of:
def calc_area(r):
pi = 3.14159
area = pi * r * r
return area
2. Use Meaningful Variable Names and Descriptive Code
Choose variable names that accurately describe what they represent. A meaningful name can make a significant difference in understanding the purpose of the code at a glance. If you’re working on complex algorithms or code paths, descriptive variable names help avoid confusion and make the logic easier to follow. This principle applies not only to variables but to functions, classes, and other code elements.
Example:
user_age = 25 # better than age = 25
user_email = 'user@example.com' # better than email = 'user@example.com'
3. Write Modular and Reusable Code
Avoid writing repetitive code by designing your software in a modular fashion. This allows you to separate concerns and reuse code when needed. Use functions, classes, and libraries where appropriate. Modular code makes it easier to maintain, test, and scale your applications. If you find yourself writing the same snippet of code multiple times, consider creating a reusable helper function or library that encapsulates that functionality.
Example:
def calculate_average(numbers):
return sum(numbers) / len(numbers)
instead of:
def calculate_average(numbers_list):
total = 0
for number in numbers_list:
total += number
return total / len(numbers_list)
4. Follow Consistent Coding Standards and Style
Consistency is key when writing code. Use a consistent style guide or coding standards, whether it’s from your company, a popular open-source project, or community consensus (like PEP 8 for Python or Google’s Java style guide). Consistent code is easier to read, maintain, and refactor. This applies to indentation, naming conventions, commenting, and overall code structure. Consistency reduces cognitive load for anyone reading your code, making it easier to understand, debug, and collaborate.
5. Avoid Premature Optimization
“Premature optimization is the root of all evil,” is a popular saying in programming. Focus first on writing clean, readable, and maintainable code before worrying about performance. Optimize only if profiling shows that performance is an issue. Modern compilers and runtime environments are highly optimized, so it’s often unnecessary to spend extra time tweaking performance prematurely.
6. Test Early and Often
Testing is an essential part of the development process, and it should be done as early and frequently as possible. Write unit tests for your code, use automated testing where feasible, and prioritize comprehensive test coverage. Testing not only validates the correctness of your code but also serves as a safeguard against regressions, making your codebase more reliable and maintainable.
7. Document Your Code Adequately
Documentation is often overlooked but incredibly valuable. Document your code so that it’s easy for others (or even future you) to understand what’s happening. This includes adding inline comments to complex parts of your code, writing clear function and class definitions, and documenting API endpoints. Good documentation can significantly speed up onboarding new team members and ensure your project remains maintainable over time.
8. Stay Open to Feedback and Collaboration
Whether you’re a novice or a seasoned developer, staying open to feedback and collaboration can greatly enhance your skills and the quality of your code. Be open to constructive criticism from peers, mentors, or code reviewers. Participate in code reviews actively and use this as an opportunity to learn and grow. Collaborating with others often brings new perspectives and ideas that can improve your solutions.
9. Refactor Wisely
Refactoring is an essential part of software development. Regularly review your code and identify opportunities for improvement, whether it’s simplifying logic, breaking up large methods, or making better use of data structures. However, be careful not to refactor purely for the sake of it—always have a reason or goal in mind, such as increasing readability or performance.
10. Use Version Control (Git) Effectively
Version control (like Git) is a vital tool for modern developers. Use commits wisely to document the evolution of your code and provide context for changes. Avoid committing large, complex changes all at once. Break up your code into smaller, logical chunks, and document your reasoning in commit messages. Good version control practices make it easier to collaborate, revert changes, and maintain a history of your project.
11. Be Mindful of Naming Conventions and Best Practices in Your Language
Each programming language has its own set of best practices and naming conventions. Familiarize yourself with these and apply them in your code. Naming variables, functions, and classes according to the conventions of the language you’re using can make your code more readable and maintainable. For example, in Python, follow PEP 8 guidelines, while in Java, adhere to the official coding style guide.
12. Code for Readability First, Performance Second
Prioritize code readability over premature optimization. Clear, readable code is easier to understand, maintain, and collaborate on, even if it runs slightly slower. The focus should be on making your code understandable and efficient, not necessarily the fastest at the moment of writing.
13. Balance Testing with Production Needs
Testing should never be neglected, but it’s important to strike a balance between comprehensive testing and delivering functionality quickly. Test early and often but understand that some aspects of a project might require quicker iterations over perfect test coverage. Find a middle ground that allows for high-quality code while delivering features promptly.
FAQ
- What are the unspoken rules of coding?
- The unspoken rules of coding include simplicity, readability, modularity, testing, and documentation. These guidelines often go beyond the technical requirements and touch on best practices that improve collaboration, maintainability, and code quality.
- Why is simplicity important in coding?
- Simplicity makes code easier to read, maintain, and debug. It avoids unnecessary complexity and makes it easier for other developers to understand your code.
- How can modular code improve maintainability?
- Modular code allows you to separate concerns and write reusable, well-defined functions or components. This makes your codebase easier to maintain and scale.
- Why should developers avoid premature optimization?
- Premature optimization can lead to inefficient code that isn’t easy to read or maintain. Modern compilers and runtime environments are optimized for performance, so focus on clean, maintainable code first.
- How important is documentation in coding?
- Documentation helps future developers understand your code. It saves time during onboarding, speeds up debugging, and makes the codebase easier to navigate.
Conclusion
The unspoken rules of coding are not hard-and-fast rules but rather best practices and standards that contribute to writing quality code, collaborating efficiently, and maintaining a healthy and productive development environment. Whether you’re a novice or an experienced developer, keeping these principles in mind can help you grow as a programmer and make a meaningful contribution to your projects. Embrace simplicity, prioritize maintainability, and always be open to learning and feedback. Happy coding!